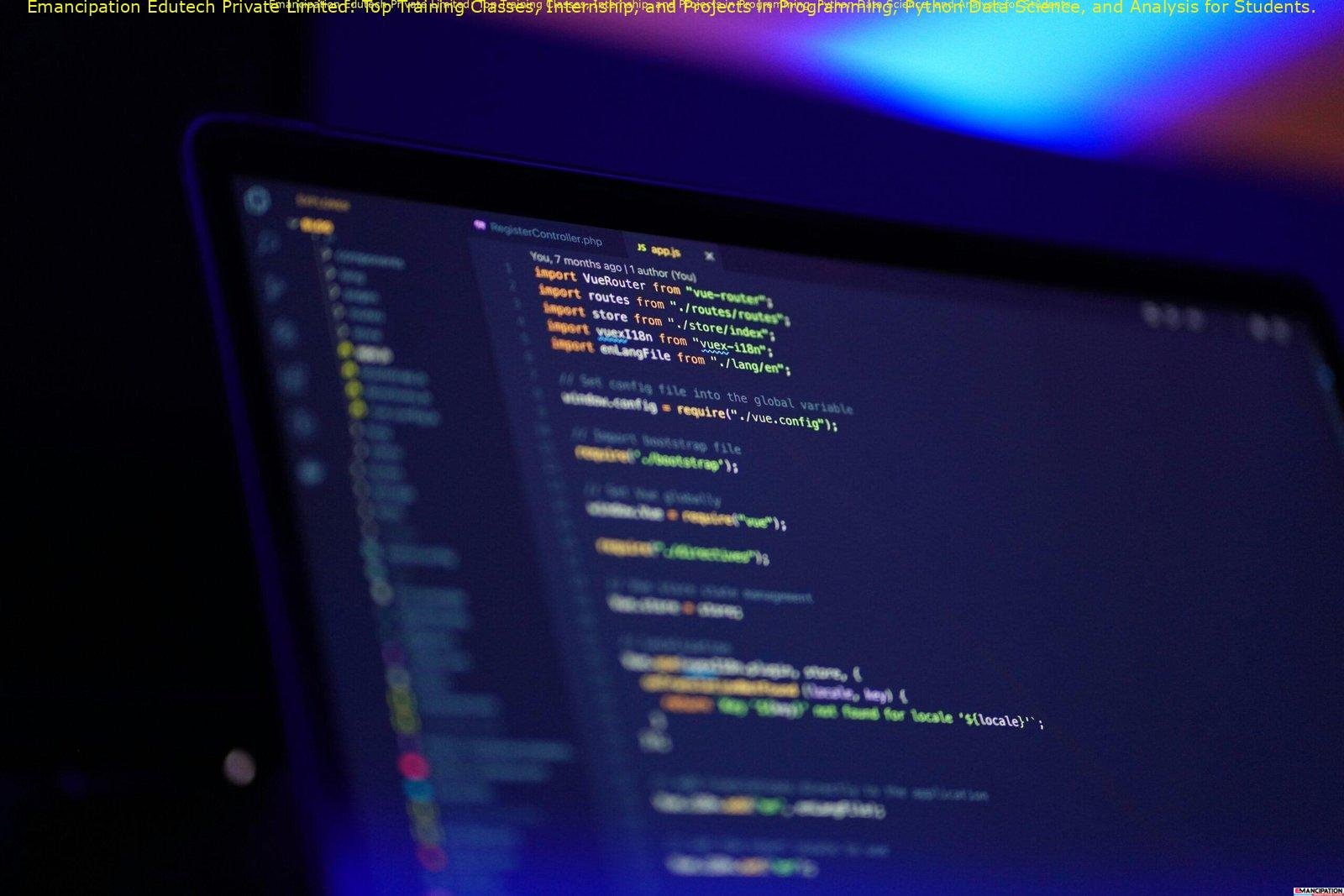
Introduction to std::vector and C-Style Arrays
In the realm of C++ programming, understanding the distinction between std::vector and traditional C-style arrays is fundamental. Both serve the purpose of storing collections of elements, but they do so in markedly different ways, reflecting their respective origins and design philosophies.
std::vector, part of the C++ Standard Library, is a template class that provides a sequence container for dynamic array management. One of its key advantages is that it can dynamically resize itself to accommodate additional elements, which offers significant flexibility during runtime. This dynamic behavior is facilitated by underlying mechanisms such as automatic memory management, which abstracts the often complex and error-prone process of manual memory allocation and deallocation in C.
On the other hand, C-style arrays originate from the C programming language, which is the predecessor of C++. These arrays are statically sized, meaning their length must be determined at the time of declaration and cannot be changed thereafter. This characteristic can lead to inefficiencies and potential memory management issues, such as buffer overflows, if not handled with care. Despite these limitations, C-style arrays are appreciated for their simplicity and direct access to memory, making them a staple in performance-critical applications.
Both std::vector and C-style arrays hold significant relevance in modern C++ programming. While std::vector is often preferred for its ease of use and safety features, C-style arrays are still prevalent in legacy systems, low-level programming, and scenarios where performance overhead must be minimized. Understanding the nuances between these two types of arrays is crucial for making informed decisions based on the specific requirements of a given application.
In the context of C++, leveraging the appropriate data structure—whether it be std::vector or a C-style array—can significantly impact the efficiency, maintainability, and robustness of the code. As we delve deeper into their individual characteristics and performance implications, it becomes evident why a solid grasp of both is indispensable for any proficient C++ programmer.
Memory Management and Allocation
Memory management and allocation constitute critical aspects when comparing std::vector and traditional C-style arrays in C++. Each has distinct characteristics that affect their usability and flexibility in various programming scenarios.
C-style arrays have a fixed size determined at compile-time. This means that once you declare a C-style array, its size cannot be altered during the program’s execution. For example, declaring an array as int arr[10];
allocates memory for 10 integers, which remains constant. While this static allocation ensures predictability, it lacks flexibility, as the array size must be known beforehand.
On the other hand, std::vector offers dynamic resizing at runtime, making it inherently more flexible. A std::vector starts with an initial capacity, which can grow as elements are added. This dynamic nature is managed internally by the vector, which automatically reallocates memory when the current capacity is exceeded. The reallocation process typically involves allocating a larger block of memory, copying the existing elements to the new block, and then freeing the old block. This is a seamless operation for the programmer, handled by the vector’s underlying implementation.
An essential component of std::vector’s memory management is the allocator. The allocator encapsulates the details of memory allocation and deallocation, providing an abstraction layer that allows for custom memory management strategies if needed. By default, std::vector uses the standard allocator, but this can be replaced with a user-defined allocator to optimize performance or memory usage for specific applications.
In summary, while C-style arrays offer straightforward and predictable memory allocation with their fixed size, std::vector provides the flexibility of dynamic resizing and sophisticated memory management, making it a more versatile choice in modern C++ programming.
Ease of Use and Flexibility
When it comes to ease of use and flexibility, std::vector stands out as a more user-friendly option compared to traditional C-style arrays. One of the primary reasons for this is the array of member functions that std::vector offers. For instance, functions like push_back and pop_back simplify the process of adding and removing elements. These operations are performed automatically, ensuring that the vector adjusts its size accordingly without requiring explicit intervention from the programmer.
In contrast, C-style arrays demand manual management, which can be both cumbersome and error-prone. For example, adding or removing an element from a C-style array necessitates shifting elements and keeping track of the array’s size manually. This not only complicates the code but also increases the likelihood of bugs and memory leaks, especially in more complex applications.
Another significant advantage of std::vector lies in its ability to provide the current size of the array through the size member function. This feature eliminates the need for auxiliary variables or functions to track the number of elements, thereby enhancing code readability and reducing potential errors. On the other hand, with C-style arrays, developers often resort to maintaining separate size variables, which can become inconsistent and lead to logical errors if not managed carefully.
The implications of these differences on code readability and maintenance are profound. std::vector‘s streamlined interface promotes cleaner, more intuitive code, making it easier for developers to understand and modify. Maintenance becomes more straightforward, as the risk of encountering low-level memory management issues is significantly reduced. Conversely, the manual oversight required with C-style arrays can make code harder to read and maintain, particularly for teams or in long-term projects.
In summary, the enhanced ease of use and flexibility offered by std::vector make it a superior choice for many applications in C++. Its built-in functionalities not only improve developer productivity but also contribute to more robust and maintainable code.
Performance Considerations
When evaluating the performance of std::vector
and C-style arrays in C++, it is crucial to consider the overhead associated with dynamic allocation and resizing, which is a significant aspect of std::vector
. Unlike C-style arrays, which have fixed sizes determined at compile-time, std::vector
offers dynamic flexibility by allowing size adjustments during runtime. This flexibility, however, comes at a cost. Each time a std::vector
exceeds its current capacity, it must allocate a new, larger memory block, copy existing elements to the new block, and then deallocate the old block. This process, known as reallocation, introduces time complexity that can impact performance, especially in scenarios involving frequent insertions and deletions.
In contrast, C-style arrays do not incur the overhead of dynamic resizing since their size is fixed. As a result, they provide deterministic performance with constant-time access and manipulation of elements. This characteristic makes C-style arrays particularly efficient for applications where the dataset size is known in advance and does not change, such as in embedded systems or real-time applications where predictability and low latency are critical.
Despite the performance advantages of C-style arrays, they lack the flexibility and safety features provided by std::vector
. For instance, std::vector
includes bounds checking, automatic memory management, and a suite of utility functions that simplify common operations. These features significantly reduce the risk of memory leaks and buffer overflows, which are common pitfalls when using C-style arrays.
Ultimately, the choice between std::vector
and C-style arrays involves a trade-off between flexibility and performance. std::vector
is well-suited for applications where dynamic resizing and ease of use are prioritized, while C-style arrays are preferable in performance-critical contexts where the overhead of dynamic memory management must be minimized. Understanding these trade-offs allows developers to make informed decisions based on the specific requirements of their projects.
Safety and Error Handling
When comparing std::vector and traditional C-style arrays in C++, it becomes evident that std::vector offers superior safety and error handling mechanisms. One of the most notable issues with C-style arrays is the risk of buffer overflows. Since C-style arrays do not inherently track their own size, programmers must manually ensure they do not exceed the array’s bounds. This can lead to dangerous memory access errors, causing unpredictable behavior or even security vulnerabilities.
In contrast, std::vector provides built-in size tracking and bounds checking. The methods at()
and operator[]
are used to access elements, but with a key difference: while operator[]
does not perform bounds checking, at()
throws an std::out_of_range
exception if an invalid index is accessed. This allows developers to handle out-of-bounds errors more gracefully and improves the robustness of the code. Moreover, std::vector’s dynamic resizing capabilities ensure that the container grows as needed, reducing the risk of buffer overflows.
Another aspect of safety in std::vector is its use of exceptions for error handling. When memory allocation fails, std::vector throws an std::bad_alloc
exception, enabling programmers to respond to memory issues appropriately. This contrasts with C-style arrays, where memory allocation failures typically result in a null pointer, necessitating explicit checks and handling by the programmer.
In modern C++ code, the use of std::vector is encouraged due to its adherence to the principles of RAII (Resource Acquisition Is Initialization). std::vector automatically manages its memory, ensuring that resources are properly allocated and deallocated without requiring explicit intervention from the programmer. This reduces the risk of memory leaks, a common issue with C-style arrays where manual deallocation is necessary.
Lastly, std::vector integrates seamlessly with C++’s standard library algorithms and iterators, which often include additional safety checks and optimizations. This further promotes the writing of safe, efficient, and maintainable code.
In summary, the safety and error handling features of std::vector make it a superior choice over traditional C-style arrays in C++. Its built-in mechanisms for bounds checking, exception handling, and automatic memory management contribute to more robust and secure C++ applications.
Interfacing with Legacy Code
Interfacing with legacy code is a common challenge for developers working in C++, particularly when transitioning from traditional C-style arrays to the modern std::vector
. Legacy systems often rely heavily on C-style arrays due to their simplicity and historical precedence. However, std::vector
offers numerous advantages such as dynamic resizing, automatic memory management, and a rich set of built-in functions. Integrating std::vector
into existing codebases that originally used C-style arrays requires a thoughtful approach to ensure compatibility and maintain the integrity of the system.
One of the primary challenges in this transition is maintaining the interface and function signatures that rely on C-style arrays. A common solution is to provide overloads for functions, accepting both std::vector
and C-style arrays. This allows legacy code to continue functioning while new code can leverage the benefits of std::vector
. For example:
void processArray(int* array, size_t size);void processArray(const std::vector& vec);
Another challenge is managing memory allocation and deallocation. C-style arrays require explicit memory management, often leading to potential memory leaks or buffer overflows. Conversely, std::vector
handles memory management automatically, reducing such risks. When converting C-style arrays to std::vector
, developers should ensure proper deallocation of dynamically allocated arrays to avoid memory leaks. This can be achieved by constructing a std::vector
directly from a pointer and size, then freeing the original array:
int* oldArray = new int[10];// Populate oldArraystd::vector vec(oldArray, oldArray + 10);delete[] oldArray;
For developers looking to transition from C-style arrays to std::vector
, it’s important to start by identifying critical sections of code where arrays are used. Gradually refactor these sections, replacing arrays with std::vector
, and thoroughly test to ensure functionality remains intact. Additionally, leveraging modern C++ features like range-based for loops, which work seamlessly with std::vector
, can simplify the transition and improve code readability.
Overall, while interfacing with legacy code comes with its set of challenges, a systematic approach to integrating std::vector
can lead to more robust and maintainable C++ codebases in the long run.
Use Cases and Practical Examples
When deciding between using std::vector
and traditional C-style arrays in C++, understanding the specific use cases and practical scenarios can guide optimal decision-making. Here, we explore various instances where each type of array excels, along with illustrative code snippets.
In scenarios involving dynamic list management, std::vector
is often the preferred choice. Unlike C-style arrays, std::vector
offers flexibility in resizing. Consider a situation where a program needs to store a list of user inputs which can grow or shrink dynamically. Using a std::vector
allows seamless addition and removal of elements:
#include <iostream>#include <vector>int main() {std::vector<int> userInputs;userInputs.push_back(10); // Adding elementsuserInputs.push_back(20);userInputs.pop_back();// Removing elements// Iterate and print elementsfor(int i : userInputs) {std::cout << i << ' ';}return 0;}
Conversely, in performance-critical applications where memory overhead must be minimized, traditional C-style arrays might be more suitable. C-style arrays offer faster access times due to the absence of additional overhead associated with automatic resizing and bounds checking. For example, in a real-time system where deterministic performance is crucial, a C-style array can be used as follows:
#include <iostream>int main() {int numbers[5] = {1, 2, 3, 4, 5};// Iterate and print elementsfor(int i = 0; i < 5; ++i) {std::cout << numbers[i] << ' ';}return 0;}
Best practices for choosing between std::vector
and C-style arrays hinge on the specific requirements of the application. If the array size is fixed and performance is critical, a C-style array may be beneficial. However, if the application requires frequent resizing or benefits from the higher-level functionalities provided by the Standard Template Library (STL), std::vector
is advisable.
In summary, both std::vector
and C-style arrays have their unique strengths and weaknesses. Understanding the specific needs of your application will help in making an informed choice, ensuring efficient and maintainable code.
Conclusion and Final Thoughts
In examining the key differences between std::vector
and traditional C-style arrays in C++, it becomes clear that each has its own set of advantages and appropriate use cases. std::vector
offers dynamic sizing, automatic memory management, and a rich set of functionalities, making it a highly versatile and developer-friendly option for many applications. This container simplifies handling dynamically sized data, reducing the burden of manual memory management and mitigating the risk of common errors such as buffer overflows.
Conversely, C-style arrays provide a lightweight alternative where performance is paramount and memory footprint needs to be minimal. Their fixed size and absence of overhead make them suitable for scenarios where resource constraints are a critical consideration, such as in embedded systems or performance-intensive applications. However, this simplicity comes at the cost of flexibility and safety, requiring developers to meticulously manage memory and array bounds.
Ultimately, the decision between using std::vector
and C-style arrays should be guided by the specific requirements of your project. For most general-purpose applications, std::vector
will likely be the preferred choice due to its ease of use and robust feature set. On the other hand, for applications where every byte and cycle count, the leaner C-style array may be more appropriate.
As you continue to develop in C++, it is crucial to evaluate the trade-offs associated with each option. Consider factors such as code readability, maintainability, performance, and safety. By doing so, you will be better equipped to choose the most suitable data structure for your needs, leveraging the strengths of both std::vector
and C-style arrays to create efficient and effective software solutions.