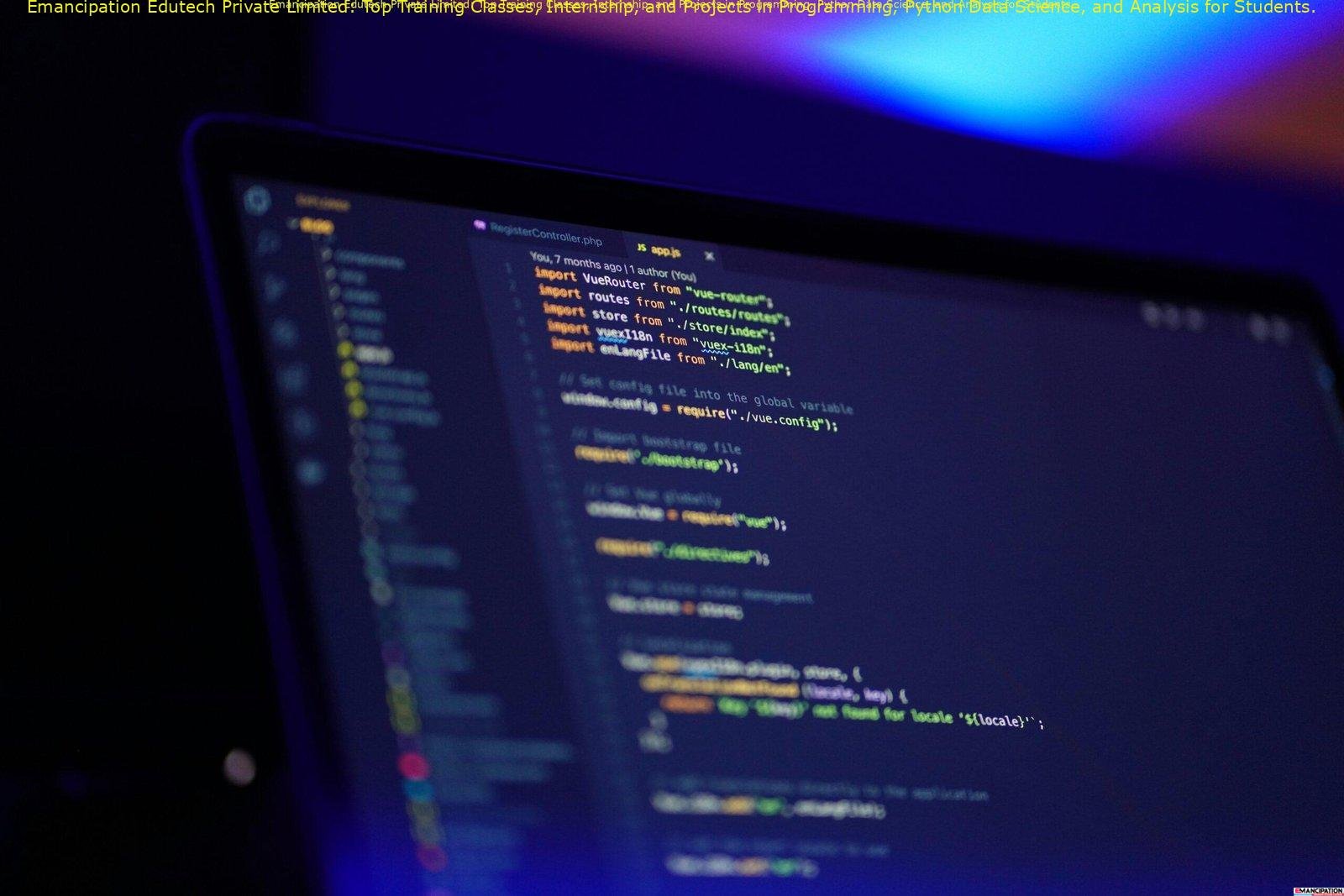
Introduction to Longest Common Subsequence (LCS)
The Longest Common Subsequence (LCS) is a fundamental concept in computer science and computational biology. It is defined as the longest sequence that can be derived from two strings by deleting some or none of their characters, without reordering the remaining characters. For instance, if we consider the strings “ABCBDAB” and “BDCAB,” the LCS would be “BCAB.” This problem is pivotal in various domains, including DNA sequencing, text comparison, and file diff tools, making it an indispensable tool for researchers and developers alike.
In DNA sequencing, identifying the LCS assists in comparing genetic sequences to find similarities and evolutionary relationships between different species. This is crucial in understanding genetic diseases and developing targeted medical treatments. Similarly, in text comparison, LCS algorithms can be employed to detect plagiarism, compare different versions of documents, or even in spell-checking tools. By identifying the common substrings, these algorithms ensure that the content remains coherent and free of unintended duplications.
File diff tools, commonly used in version control systems, also rely on the LCS to highlight changes between different versions of a file. Developers use these tools to track modifications, manage code versions, and collaborate more effectively on software projects. By visualizing the differences and commonalities in code, LCS algorithms help maintain the integrity and consistency of software applications.
To illustrate the concept further, consider two strings: “XMJYAUZ” and “MZJAWXU.” The LCS for these strings is “MJAU.” This demonstrates how the LCS can be identified despite the presence of non-matching characters and varying string lengths. Understanding and implementing the LCS algorithm in Python can significantly enhance your ability to handle complex string comparison tasks efficiently.
Understanding the Problem Statement
In Python programming, one often encounters the need to analyze and compare sequences of data. A common problem in this realm is finding the Longest Common Subsequence (LCS) between two given strings. The LCS is defined as the longest sequence that appears in both strings in the same order, but not necessarily contiguously. Understanding this distinction is crucial for implementing an effective solution.
To elucidate the difference between a subsequence and a substring, consider the strings “Python” and “Ranchi”. A substring is a contiguous sequence of characters within a string. For instance, “Pyt” is a substring of “Python”. Conversely, a subsequence does not require characters to be contiguous, as long as they appear in the same order. Therefore, “Ptn” is a subsequence of “Python”.
The problem of finding the LCS involves identifying the longest sequence of characters that appears in the same order in both strings. For example, given the strings “Python” and “Ranchi”, the LCS is “hn”. This is because “hn” is the longest sequence that can be observed in both strings while preserving the order of appearance.
To further clarify, consider the strings “AGGTAB” and “GXTXAYB”. The LCS for these strings is “GTAB”, as it appears in both strings in the same order. The process of identifying the LCS typically involves dynamic programming due to its efficiency in solving overlapping subproblems and storing intermediate results.
Understanding the problem statement is the first step towards implementing a Python function to find the LCS. By leveraging Python’s powerful data structures and control flow mechanisms, one can efficiently address this problem, producing a solution that is both elegant and effective. Through this blog post, we will delve deeper into the methodologies and Python code necessary to achieve this objective.
The dynamic programming approach to solving the Longest Common Subsequence (LCS) problem is one of the most efficient methods available. This approach hinges on the idea of breaking down the larger problem into smaller, manageable subproblems and then storing the results of these subproblems to avoid redundant calculations. This technique, known as “memoization,” is fundamental in optimizing the solution and reducing computational overhead.
To implement this approach, we utilize a two-dimensional table, often referred to as a DP table (Dynamic Programming table). The DP table helps in storing intermediate results, thereby enabling us to build the solution incrementally. The DP table is essentially a matrix where the cell at position (i, j) contains the length of the LCS of the substrings X[0…i-1] and Y[0…j-1]. This matrix aids in visualizing and computing the solution more systematically.
The construction of the DP table involves initializing the first row and the first column to zeros. This initialization represents the base cases where if one string is empty, the LCS length is zero. Once initialized, the table is filled using the following recurrence relation:
If the characters of the two strings match, i.e., X[i-1] == Y[j-1], then:
DP[i][j] = DP[i-1][j-1] + 1
If the characters do not match, we take the maximum value from the adjacent cells:
DP[i][j] = max(DP[i-1][j], DP[i][j-1])
This process continues until the entire DP table is filled. The value in the bottom-right cell of the table, DP[m][n], will then represent the length of the LCS of the given two strings X of length m and Y of length n. This method ensures an optimal time complexity of O(m*n), making it highly suitable for practical applications involving the Python programming language, particularly in Ranchi, where Python’s usage is growing rapidly among developers and educators.
In the realm of dynamic programming, defining a DP table is a critical step in solving complex problems efficiently. When it comes to finding the Longest Common Subsequence (LCS) between two strings using Python, the DP table serves as the cornerstone of our approach. The DP table, also known as a matrix, is a two-dimensional array where each cell represents a subproblem’s solution.
The dimensions of the DP table are determined by the lengths of the two input strings. Suppose we have two strings, A and B, with lengths m and n respectively. Our DP table will have dimensions (m+1) x (n+1). The extra row and column are used to handle the base cases where one of the strings is empty. Each cell dp[i][j] in this table will store the length of the LCS of the substrings A[0..i-1] and B[0..j-1].
The recurrence relation is the formula that allows us to fill in the DP table based on previously computed values. It is derived from the problem’s requirements and the nature of the LCS. The relation can be stated as follows:
1. If A[i-1] == B[j-1], then dp[i][j] = dp[i-1][j-1] + 1. This indicates that the characters match, so we extend the LCS found so far by one.
2. If A[i-1] != B[j-1], then dp[i][j] = max(dp[i-1][j], dp[i][j-1]). This suggests that we either exclude the character from A or B and take the maximum of the two possible LCS lengths.
3. For the base cases, dp[i][0] = 0 for all i and dp[0][j] = 0 for all j. This is because the LCS of any string with an empty string is always zero.
By systematically filling out this table using the recurrence relation, we can efficiently compute the length of the longest common subsequence. This approach leverages the strengths of Python’s array handling capabilities to manage and populate the DP table effectively, ensuring optimal performance even for longer strings.
Implementing the LCS Function in Python
To implement a function in Python that finds the Longest Common Subsequence (LCS) between two strings, we begin by initializing a dynamic programming (DP) table. This table will help us store the lengths of LCSs for substrings of the given strings. The DP table is a two-dimensional array where the cell at index [i][j]
contains the length of the LCS of the substrings ending at i
and j
.
First, we initialize the DP table with zeros. The dimensions of the table will be (m+1) x (n+1)
, where m
and n
are the lengths of the two strings. The extra row and column are used to handle the base case where one of the strings is empty:
def lcs(X, Y):m = len(X)n = len(Y)dp = [[0] * (n + 1) for _ in range(m + 1)]
Next, we fill the DP table using nested loops. The outer loop iterates through each character of the first string, and the inner loop iterates through each character of the second string. For every pair of characters X[i-1]
and Y[j-1]
, we apply the following recurrence relation:
- If
X[i-1] == Y[j-1]
, thendp[i][j] = dp[i-1][j-1] + 1
- Otherwise,
dp[i][j] = max(dp[i-1][j], dp[i][j-1])
This recurrence relation ensures that if the characters match, the length of the LCS is incremented by one. If they do not match, the length of the LCS is the maximum length found by excluding one of the characters:
for i in range(1, m + 1):for j in range(1, n + 1):if X[i - 1] == Y[j - 1]:dp[i][j] = dp[i - 1][j - 1] + 1else:dp[i][j] = max(dp[i - 1][j], dp[i][j - 1])
Finally, the value at dp[m][n]
will be the length of the longest common subsequence of the two strings. We can return this value as the result:
return dp[m][n]
Putting it all together, we have:
def lcs(X, Y):m = len(X)n = len(Y)dp = [[0] * (n + 1) for _ in range(m + 1)]for i in range(1, m + 1):for j in range(1, n + 1):if X[i - 1] == Y[j - 1]:dp[i][j] = dp[i - 1][j - 1] + 1else:dp[i][j] = max(dp[i - 1][j], dp[i][j - 1])return dp[m][n]
This Python function effectively computes the LCS of two given strings using a dynamic programming approach. This method ensures optimal performance and clarity, making it a valuable algorithm for various applications in Ranchi and beyond.
Extracting the LCS from the DP Table
With the Dynamic Programming (DP) table fully populated, the next step in determining the Longest Common Subsequence (LCS) between two strings is to extract it by tracing back from the bottom-right corner of the table. This process involves a systematic traversal of the table to reconstruct the LCS string.
The bottom-right cell of the DP table contains the length of the LCS. To trace back and extract the LCS, we start from this cell and move towards the top-left corner, following specific rules that guide us through the optimal path. If we denote the two strings as `X` and `Y`, and the DP table as `dp`, the process can be described as follows:
1. Initialize an empty list to store the LCS characters.2. Set pointers `i` and `j` to point to the last characters of `X` and `Y`, respectively.3. While both `i` and `j` are greater than zero:- If `X[i-1]` is equal to `Y[j-1]`, this means the characters match and are part of the LCS. Append `X[i-1]` to the list and move diagonally up-left to `dp[i-1][j-1]`.- If the characters do not match, move in the direction of the cell with the larger value: either up to `dp[i-1][j]` or left to `dp[i][j-1]`.4. Reverse the list to get the LCS in the correct order.
The following Python code snippet illustrates this traceback process:
def extract_lcs(dp, X, Y):i, j = len(X), len(Y)lcs = []while i > 0 and j > 0:if X[i-1] == Y[j-1]:lcs.append(X[i-1])i -= 1j -= 1elif dp[i-1][j] > dp[i][j-1]:i -= 1else:j -= 1lcs.reverse()return ''.join(lcs)# Example usage:X = "ABCBDAB"Y = "BDCAB"dp = [[0, 0, 0, 0, 0, 0], [0, 0, 0, 1, 1, 1], [0, 0, 1, 1, 1, 2], [0, 1, 1, 2, 2, 2], [0, 1, 1, 2, 3, 3], [0, 1, 2, 2, 3, 4], [0, 1, 2, 3, 3, 4], [0, 1, 2, 3, 4, 4]]print(extract_lcs(dp, X, Y))# Output: "BCAB"
This code accurately follows the traceback method, ensuring the correct reconstruction of the LCS. Understanding this process is crucial for implementing robust solutions in Python, particularly in applications where sequence alignment or comparison is essential, such as bioinformatics or text processing in Ranchi or elsewhere.
When implementing a function to find the Longest Common Subsequence (LCS) between two strings in Python, optimizing the function can lead to significant improvements in both performance and resource utilization. One effective approach to optimization involves reducing the space complexity of the function by using only two rows of the dynamic programming (DP) table, rather than maintaining the entire matrix.
In the original LCS algorithm, a 2D table of size m x n (where m and n are the lengths of the two input strings) is used to store the lengths of LCSs for all substrings. This results in a space complexity of O(mn). While this approach is straightforward, it can be memory-intensive, especially for longer strings.
To optimize the space complexity, we can utilize a rolling array technique. Instead of storing the entire DP table, we maintain only two rows at any given time: the current row and the previous row. As we iterate through the characters of the strings, we update these two rows accordingly. This reduces the space complexity from O(mn) to O(n), where n is the length of the shorter string.
Here’s a brief look at how this optimization works:
1. Initialize two arrays, prev
and curr
, each of size n+1.2. Iterate over the characters of the first string.3. For each character in the first string, iterate over the characters of the second string.4. Update the curr
array based on the values in the prev
array and the current characters of both strings.5. After processing each character of the first string, swap the prev
and curr
arrays.
This approach maintains the same time complexity of O(mn) as the original algorithm, since we still process each character pair once. However, by reducing the space complexity, it becomes more efficient in terms of memory usage.
In conclusion, optimizing the LCS function in Python by using a rolling array technique can lead to significant improvements in space efficiency without compromising the time complexity. This makes the algorithm more suitable for applications involving long strings or constrained memory environments, enhancing its overall practicality and performance.
Testing and Validating the LCS Function
Ensuring the reliability and accuracy of the Longest Common Subsequence (LCS) function necessitates rigorous testing. This process involves creating a diverse set of test cases, which will comprehensively evaluate the function’s performance across different scenarios. By methodically testing the LCS function, you can confirm its robustness and correctness, thereby enhancing its practical utility in various applications.
To initiate the testing phase, consider a range of test cases with varying string lengths and characters. For instance, test cases might include short strings, long strings, strings with special characters, and strings with mixed cases. Such diversity ensures that the function can handle a wide array of input types.
Edge cases represent another critical aspect of testing. These include scenarios where the input strings are empty, completely disjoint, or contain repeated characters. By addressing these edge cases, you can verify that the LCS function operates correctly under all possible conditions.
Here is a sample set of test cases along with the expected outputs:
Test Case 1:
Input: str1 = “AGGTAB”, str2 = “GXTXAYB”
Expected Output: 4 (LCS: “GTAB”)
Test Case 2:
Input: str1 = “ABCDGH”, str2 = “AEDFHR”
Expected Output: 3 (LCS: “ADH”)
Test Case 3:
Input: str1 = “”, str2 = “ABC”
Expected Output: 0 (LCS: “”)
Test Case 4:
Input: str1 = “ABC”, str2 = “DEF”
Expected Output: 0 (LCS: “”)
Test Case 5:
Input: str1 = “AAB”, str2 = “AZB”
Expected Output: 2 (LCS: “AB”)
Sample test code in Python to validate the LCS function:
def test_lcs():assert lcs("AGGTAB", "GXTXAYB") == 4assert lcs("ABCDGH", "AEDFHR") == 3assert lcs("", "ABC") == 0assert lcs("ABC", "DEF") == 0assert lcs("AAB", "AZB") == 2print("All test cases passed!")test_lcs()
By conducting these tests and validating the outputs, you can ascertain the LCS function’s accuracy. Such thorough testing is indispensable for developers in Ranchi and beyond, who seek to implement reliable Python solutions in their projects. Remember, the goal is not only to achieve correct results but also to ensure that the function can handle a broad spectrum of inputs gracefully.