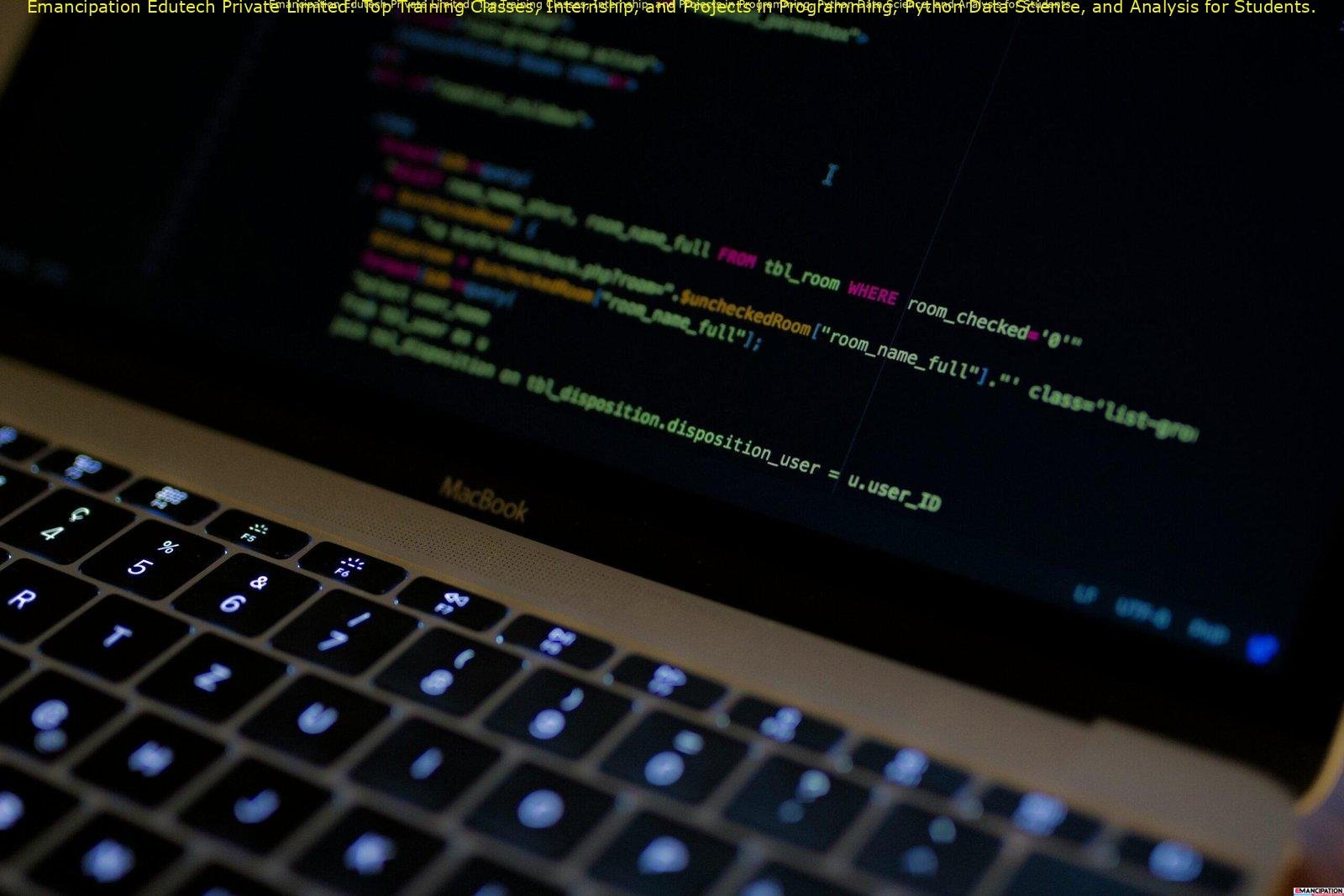
In the realm of Java, ensuring thread safety while handling collections is a critical aspect of concurrent programming. Two prominent solutions offered by the Java standard library for managing synchronized collections are ConcurrentHashMap and Collections.synchronizedMap. Both play pivotal roles in making collections thread-safe, but they achieve this objective through different mechanisms and design philosophies.
ConcurrentHashMap, introduced in Java 1.5, is a part of the java.util.concurrent package. It is specifically designed to handle concurrency more efficiently by leveraging a sophisticated locking mechanism known as lock striping. This mechanism allows multiple threads to read and write to different segments of the map concurrently, significantly reducing contention and improving throughput in highly concurrent environments. ConcurrentHashMap is ideal for situations where high read and write throughput is essential, such as in web servers or large-scale data processing applications.
On the other hand, Collections.synchronizedMap is a method provided by the java.util.Collections class to wrap a regular Map with synchronized methods. This wrapper ensures that each method call is thread-safe by synchronizing on the map object itself. While this approach guarantees thread safety, it can lead to performance bottlenecks under high contention, as only one thread can access the map at a time. Collections.synchronizedMap is more straightforward and can be suitable for less concurrent scenarios or for smaller maps where performance is not a critical factor.
By understanding the core purposes and functionalities of ConcurrentHashMap and Collections.synchronizedMap, developers can make informed decisions about which synchronization strategy to employ based on the specific requirements of their Java applications. The subsequent sections of this blog post will delve deeper into the performance characteristics, use cases, and best practices associated with each of these synchronization mechanisms.
Thread-Safety Mechanisms
When working with concurrent Java applications, ensuring thread safety is paramount. Both ConcurrentHashMap and Collections.synchronizedMap provide mechanisms to handle thread safety, but they employ different strategies that significantly impact their performance and scalability.
ConcurrentHashMap is designed for high concurrency and scalability. It achieves this through a technique known as lock stripping. In essence, lock stripping involves dividing the map into segments and each segment has its own lock. This allows multiple threads to read and write to different segments of the map simultaneously, thereby reducing contention and improving throughput. For instance, if a thread is writing to one segment, other threads can still access other segments without waiting for the write operation to complete. This granular locking mechanism ensures that operations on ConcurrentHashMap are more efficient and scalable, particularly in environments with a high degree of concurrency.
On the other hand, Collections.synchronizedMap takes a simpler approach by synchronizing all methods of the map. This means that any operation on the map, whether it is a read or a write, requires acquiring a single lock for the entire map. While this ensures thread safety, it can lead to significant contention and reduced performance, especially in scenarios with frequent read and write operations. The global lock can become a bottleneck, limiting the scalability of Collections.synchronizedMap in a multi-threaded environment.
In summary, the choice between ConcurrentHashMap and Collections.synchronizedMap largely depends on the specific requirements of your application. If your application demands high concurrency and scalability, ConcurrentHashMap is likely the better choice due to its lock stripping mechanism. However, for simpler use cases with less stringent performance requirements, Collections.synchronizedMap may suffice. By understanding the underlying thread-safety mechanisms, developers can make informed decisions to optimize their Java applications effectively.
Performance and Scalability
When evaluating performance and scalability, it becomes evident that ConcurrentHashMap and Collections.synchronizedMap offer distinct characteristics tailored to different levels of concurrent access. ConcurrentHashMap is specifically designed to handle high concurrency with minimal contention, thus making it an excellent choice for environments requiring extensive parallelism. This map employs a fine-grained locking mechanism, known as lock striping, which segments the map into multiple smaller sections. As a result, multiple threads can operate on different segments concurrently, significantly reducing the likelihood of thread contention and enhancing throughput.
On the other hand, Collections.synchronizedMap employs a single lock mechanism that guards the entire map. This approach can lead to performance bottlenecks in scenarios where numerous threads are attempting to access or modify the map simultaneously. The single lock becomes a point of contention, serializing access and effectively reducing the benefits of multithreaded execution. This makes Collections.synchronizedMap less suitable for applications experiencing heavy concurrent access.
For developers in Java, particularly those working on projects in highly competitive environments like tech hubs such as Ranchi, understanding these differences is crucial. The ability to leverage ConcurrentHashMap‘s sophisticated concurrency control can lead to more scalable and efficient applications. By minimizing lock contention and maximizing parallelism, ConcurrentHashMap ensures that applications can scale gracefully as the number of concurrent threads increases.
Conversely, while Collections.synchronizedMap may be sufficient for applications with low to moderate concurrency demands, it is not the optimal choice for high-performance, scalable systems. The potential for thread contention and the inherent limitations of a single lock mechanism make it less appropriate for environments where high concurrency is a key requirement.
Ultimately, selecting between ConcurrentHashMap and Collections.synchronizedMap should be guided by the specific performance and scalability needs of the application. For high-concurrency scenarios, the former is the clear choice, offering superior performance and the ability to handle extensive parallelism efficiently.
Usage Scenarios and Examples
When deciding between ConcurrentHashMap and Collections.synchronizedMap, understanding their usage scenarios can guide you to make the appropriate choice. Both maps are designed to handle concurrency in Java, but they excel in different contexts.
ConcurrentHashMap is optimized for scenarios involving high concurrency, where there are frequent reads and writes by multiple threads. This map divides its data into segments, allowing concurrent access to different segments, which significantly reduces contention. Let’s consider an example where a web application logs user activities that need to be processed by multiple threads:
import java.util.concurrent.ConcurrentHashMap;public class ConcurrentLogging {private ConcurrentHashMap<String, Integer> activityLog = new ConcurrentHashMap<>();public void logActivity(String user) {activityLog.merge(user, 1, Integer::sum);}public int getActivityCount(String user) {return activityLog.getOrDefault(user, 0);}}
In this scenario, the ConcurrentHashMap
allows multiple threads to log user activities without causing thread contention, ensuring that the application remains performant even under heavy load.
On the other hand, Collections.synchronizedMap is suitable for scenarios with lower concurrency requirements. It synchronizes the entire map, making it thread-safe but potentially leading to higher contention and reduced performance when accessed by multiple threads. Here’s an example illustrating its use in a simple application where concurrency is less intensive:
import java.util.Collections;import java.util.HashMap;import java.util.Map;public class SynchronizedLogging {private Map<String, Integer> activityLog = Collections.synchronizedMap(new HashMap<>());public synchronized void logActivity(String user) {activityLog.put(user, activityLog.getOrDefault(user, 0) + 1);}public synchronized int getActivityCount(String user) {return activityLog.getOrDefault(user, 0);}}
In this example, Collections.synchronizedMap
ensures that only one thread can access the map at a time, which is adequate for applications where updates are infrequent or the number of threads is limited.
Understanding the differences between ConcurrentHashMap
and Collections.synchronizedMap
allows developers to choose the right tool for their specific requirements, ensuring optimal performance and thread safety in their Java applications.
Implementation Differences
The primary distinction between ConcurrentHashMap and Collections.synchronizedMap lies in their underlying implementations, which significantly influence their behavior and performance. Understanding these differences is crucial for making informed decisions in concurrent programming, especially when working with Java in various environments, including dynamic development hubs like Ranchi.
ConcurrentHashMap is designed with high concurrency in mind. It achieves this by employing a segmented architecture. The map is divided into multiple segments, each of which can be independently locked. This segmentation allows multiple threads to operate on different segments simultaneously without contention, thereby improving throughput. Each segment is essentially a smaller version of a hash table, complete with its own lock. When a thread accesses a particular key, it only locks the corresponding segment, leaving other segments accessible to other threads.
On the other hand, Collections.synchronizedMap takes a more straightforward approach by wrapping a regular map, such as a HashMap, with synchronized methods. This ensures that every method call is thread-safe by using a single lock for the entire map. While this guarantees atomicity of operations, it also means that only one thread can access the map at any given time. This approach can lead to significant contention and reduced performance under high concurrency conditions.
These implementation differences directly impact the performance characteristics of the two collections. ConcurrentHashMap is more scalable and performs better in multi-threaded environments because it minimizes contention through its segmented locking mechanism. In contrast, Collections.synchronizedMap can become a bottleneck in scenarios with high thread contention due to its single lock strategy.
Choosing between ConcurrentHashMap and Collections.synchronizedMap should be based on the specific concurrency requirements of your application. For highly concurrent environments, especially in complex Java projects, the advanced locking mechanisms of ConcurrentHashMap offer a more efficient solution. Conversely, for simpler use cases with lower concurrency demands, Collections.synchronizedMap provides a straightforward, albeit less performant, thread-safe option.
When evaluating ConcurrentHashMap and Collections.synchronizedMap, it is essential to consider their respective advantages and disadvantages. Each has unique strengths and potential drawbacks that make them suitable for different scenarios in Java development, including Java in Ranchi, where diverse application requirements often arise.
ConcurrentHashMap
The primary advantage of ConcurrentHashMap lies in its superior performance in highly concurrent environments. It achieves this by allowing concurrent read and write operations without locking the entire map, thus minimizing contention among threads. This makes it particularly effective in multi-threaded applications where high throughput is critical. Additionally, ConcurrentHashMap provides better scalability, which is beneficial for applications experiencing high levels of concurrent access.
However, ConcurrentHashMap also has its disadvantages. Its internal complexity can make debugging and maintenance more challenging compared to simpler synchronized collections. Developers need to be aware of the nuances of its behavior, especially regarding atomicity and visibility guarantees. This can increase the learning curve for teams less familiar with concurrent programming in Java.
Collections.synchronizedMap
On the other hand, Collections.synchronizedMap offers simplicity and ease of use, making it an attractive option for projects where developer familiarity and quick implementation are priorities. It wraps a regular map in synchronized methods, providing straightforward thread safety without requiring deep knowledge of concurrency principles. This can enhance code maintainability, as its behavior is more predictable and easier to understand.
However, the main drawback of Collections.synchronizedMap is its performance under high contention. Since it locks the entire map for every read and write operation, it can become a bottleneck in applications with many concurrent threads. This limitation can significantly degrade performance, especially in scenarios demanding high concurrency levels.
In conclusion, choosing between ConcurrentHashMap and Collections.synchronizedMap depends on the specific requirements of the Java application. For high-performance, scalable systems with heavy concurrency, ConcurrentHashMap is often the better choice. Conversely, for simpler applications where ease of use and maintainability are crucial, Collections.synchronizedMap may be more appropriate. Understanding these trade-offs is vital for making informed decisions in Java development, whether in Ranchi or any other context.
Common Pitfalls and Best Practices
When working with concurrent collections in Java, particularly ConcurrentHashMap and Collections.synchronizedMap, developers often encounter various pitfalls. Understanding these can help mitigate risks and improve the efficiency of your multi-threaded applications.
One common mistake is assuming that Collections.synchronizedMap provides the same level of concurrency as ConcurrentHashMap. While Collections.synchronizedMap synchronizes access to the entire map, it can become a bottleneck in highly concurrent environments. In contrast, ConcurrentHashMap uses a more granular locking mechanism, allowing better concurrency and performance.
Another frequent issue is improper handling of null values. Unlike Collections.synchronizedMap, ConcurrentHashMap does not permit null keys or values. Attempting to insert nulls into a ConcurrentHashMap will result in a NullPointerException. This design choice helps avoid ambiguities in concurrent scenarios where the presence or absence of a key might signify different states.
To choose between these two, consider the concurrency requirements of your application. For environments with high read and write operations, ConcurrentHashMap is usually the better choice due to its scalable performance. On the other hand, Collections.synchronizedMap might suffice for simpler, less concurrent use cases.
When implementing these maps, always be mindful of the synchronization context. For instance, any composite operations, such as checking and inserting if absent, should be performed within a synchronized block when using Collections.synchronizedMap to avoid race conditions. Conversely, ConcurrentHashMap provides atomic operations like putIfAbsent, which can be used to simplify code and improve safety.
Finally, avoid excessive synchronization, which can lead to performance degradation. Profiling your Java application in Ranchi or elsewhere can help identify bottlenecks and optimize synchronization strategies. By adhering to these best practices, you can leverage the full potential of ConcurrentHashMap and Collections.synchronizedMap, ensuring efficient and robust multi-threaded applications.
Conclusion
In conclusion, understanding the key differences between ConcurrentHashMap and Collections.synchronizedMap is crucial for developers aiming to implement thread-safe operations in Java. Both classes serve the purpose of enabling concurrent access to a map but do so in fundamentally different ways. ConcurrentHashMap provides a highly concurrent, scalable, and efficient approach by segmenting the map into smaller portions that can be accessed by multiple threads simultaneously. On the other hand, Collections.synchronizedMap employs a simplistic synchronization mechanism by locking the entire map, which can lead to bottlenecks in a multi-threaded environment.
Choosing between these two options largely depends on the specific requirements of your application. If your use case demands high levels of concurrency and performance, ConcurrentHashMap would be the preferred choice due to its non-blocking nature and finer-grained locking. However, if your application involves relatively low concurrency or simpler synchronization needs, Collections.synchronizedMap might suffice, offering simplicity and ease of use.
For developers working in Java, especially those dealing with concurrent programming, deepening your understanding of these collections and their behaviors is invaluable. Further reading on Java concurrency can enhance your knowledge and help you make more informed decisions when designing thread-safe applications. Resources such as the Java Concurrency in Practice book, online tutorials, and official Java documentation are excellent starting points.
By comprehending the distinctions and appropriate use cases for ConcurrentHashMap and Collections.synchronizedMap, you can optimize your application’s performance and reliability, ensuring a robust and efficient system. For those in Ranchi or elsewhere looking to further their expertise in Java, consider engaging with local tech communities or participating in Java development forums to exchange knowledge and experiences.