1. Simple Line Plot
Python
import matplotlib.pyplot as plt
# Sample data
days = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun']
temperatures = [20, 22, 23, 21, 25, 24, 20]
# Creating the plot
plt.plot(days, temperatures)
# Adding titles and labels
plt.title('Temperature Over the Week')
plt.xlabel('Day')
plt.ylabel('Temperature (°C)')
# Save the plot
plt.savefig('line_plot.png', dpi=300, bbox_inches='tight')
plt.show()
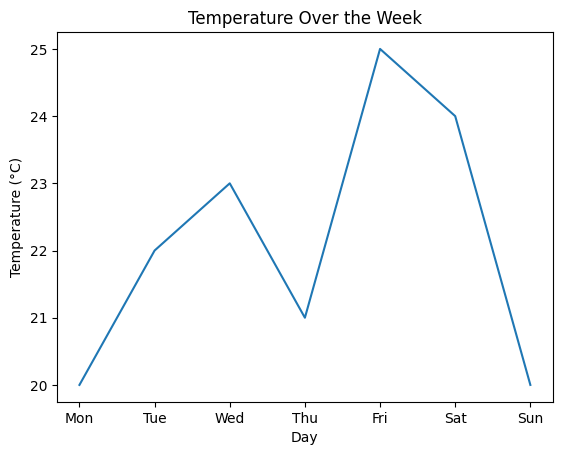
2. Customized Line Plot
Python
import matplotlib.pyplot as plt
# Sample data
days = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun']
temperatures = [20, 22, 23, 21, 25, 24, 20]
# Creating the plot with customization
plt.plot(days, temperatures, color='purple', marker='o', linestyle='--')
# Adding titles and labels
plt.title('Temperature Over the Week', fontsize=16)
plt.xlabel('Day', fontsize=12)
plt.ylabel('Temperature (°C)', fontsize=12)
# Save the plot
plt.savefig('customized_line_plot.png', dpi=300, bbox_inches='tight')
plt.show()

3. Plotting Multiple Lines
Python
import matplotlib.pyplot as plt
# Sample data
days = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun']
temperatures = [20, 22, 23, 21, 25, 24, 20]
temperatures_last_week = [19, 21, 20, 22, 24, 22, 19]
# Creating the plot with two lines
plt.plot(days, temperatures, color='purple', marker='o', linestyle='--', label='This Week')
plt.plot(days, temperatures_last_week, color='orange', marker='x', linestyle='-', label='Last Week')
# Adding titles, labels, and legend
plt.title('Temperature Comparison Over Weeks', fontsize=16)
plt.xlabel('Day', fontsize=12)
plt.ylabel('Temperature (°C)', fontsize=12)
plt.legend()
# Save the plot
plt.savefig('multiple_lines_plot.png', dpi=300, bbox_inches='tight')
plt.show()

4. Scatter Plot
Python
import matplotlib.pyplot as plt
# Sample data
hours_studied = [1, 2, 3, 4, 5, 6, 7, 8, 9]
test_scores = [50, 55, 60, 65, 70, 75, 80, 85, 90]
# Creating a scatter plot
plt.scatter(hours_studied, test_scores, color='green', marker='s')
# Adding titles and labels
plt.title('Relationship Between Study Hours and Test Scores')
plt.xlabel('Hours Studied')
plt.ylabel('Test Score')
# Save the plot
plt.savefig('scatter_plot.png', dpi=300, bbox_inches='tight')
plt.show()

5. Bar Plot
Python
import matplotlib.pyplot as plt
# Sample data
products = ['A', 'B', 'C', 'D']
sales = [250, 300, 200, 400]
# Creating a bar plot
plt.bar(products, sales, color='skyblue')
# Adding titles and labels
plt.title('Sales by Product')
plt.xlabel('Product')
plt.ylabel('Sales (units)')
# Save the plot
plt.savefig('bar_plot.png', dpi=300, bbox_inches='tight')
plt.show()

6. Histogram
Python
import matplotlib.pyplot as plt
# Sample data
ages = [22, 21, 25, 30, 32, 35, 28, 22, 25, 30, 40, 42, 34, 36, 38]
# Creating a histogram
plt.hist(ages, bins=5, color='coral', edgecolor='black')
# Adding titles and labels
plt.title('Age Distribution')
plt.xlabel('Age')
plt.ylabel('Frequency')
# Save the plot
plt.savefig('histogram.png', dpi=300, bbox_inches='tight')
plt.show()

7. Custom Colors and Styles
Python
import matplotlib.pyplot as plt
# Sample data
days = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun']
temperatures = [20, 22, 23, 21, 25, 24, 20]
# Creating a line plot with custom colors
plt.plot(days, temperatures, color='#FF5733', marker='o', linestyle='-', label='This Week')
# Adding titles and labels
plt.title('Temperature Over the Week', fontsize=16, color='darkblue')
plt.xlabel('Day', fontsize=12, color='darkblue')
plt.ylabel('Temperature (°C)', fontsize=12, color='darkblue')
# Adding grid
plt.grid(True, which='both', linestyle='--', linewidth=0.5)
# Save the plot
plt.savefig('custom_colors_styles.png', dpi=300, bbox_inches='tight')
plt.show()

8. Annotations
Python
import matplotlib.pyplot as plt
# Sample data
days = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun']
temperatures = [20, 22, 23, 21, 25, 24, 20]
# Creating a line plot with annotation
plt.plot(days, temperatures, color='purple', marker='o', linestyle='--')
# Adding annotation
plt.annotate('Highest Temp', xy=('Fri', 25), xytext=('Sat', 23), arrowprops=dict(facecolor='black', shrink=0.05))
# Adding titles and labels
plt.title('Temperature Over the Week')
plt.xlabel('Day')
plt.ylabel('Temperature (°C)')
# Save the plot
plt.savefig('annotations.png', dpi=300, bbox_inches='tight')
plt.show()

9. Subplots
Python
import matplotlib.pyplot as plt
# Sample data
days = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun']
temperatures = [20, 22, 23, 21, 25, 24, 20]
temperatures_last_week = [19, 21, 20, 22, 24, 22, 19]
products = ['A', 'B', 'C', 'D']
sales = [250, 300, 200, 400]
# Creating subplots
fig, ax = plt.subplots(1, 2, figsize=(10, 5))
# First subplot
ax[0].plot(days, temperatures, color='purple', marker='o', linestyle='--')
ax[0].set_title('This Week')
# Second subplot
ax[1].bar(products, sales, color='skyblue')
ax[1].set_title('Sales by Product')
# Overall titles and labels
plt.suptitle('Temperature and Sales Comparison')
# Save the plot
plt.savefig('subplots.png', dpi=300, bbox_inches='tight')
plt.show()

10. 3D Plot
Python
from mpl_toolkits.mplot3d import Axes3D
import numpy as np
import matplotlib.pyplot as plt
# Creating data
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X**2 + Y**2))
# Creating a 3D plot
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(X, Y, Z, cmap='viridis')
# Adding titles and labels
ax.set_title('3D Surface Plot')
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
# Save the plot
plt.savefig('3d_surface_plot.png', dpi=300, bbox_inches='tight')
plt.show()

11. Animated Plot
Python
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
# Creating a figure and axis
fig, ax = plt.subplots()
# Setting up the plot
x = np.linspace(0, 2*np.pi, 100)
line, = ax.plot(x, np.sin(x))
# Animation function
def animate(i):
line.set_ydata(np.sin(x + i/10))
return line,
# Creating the animation
ani = animation.FuncAnimation(fig, animate, frames=100, interval=20, blit=True)
# Save the animation
ani.save('animated_sine_wave.gif', writer='imagemagick')
# Display the animation
plt.show()
WARNING:matplotlib.animation:MovieWriter imagemagick unavailable; using Pillow instead.
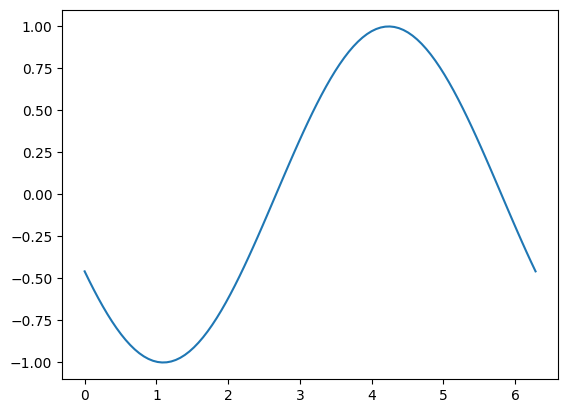