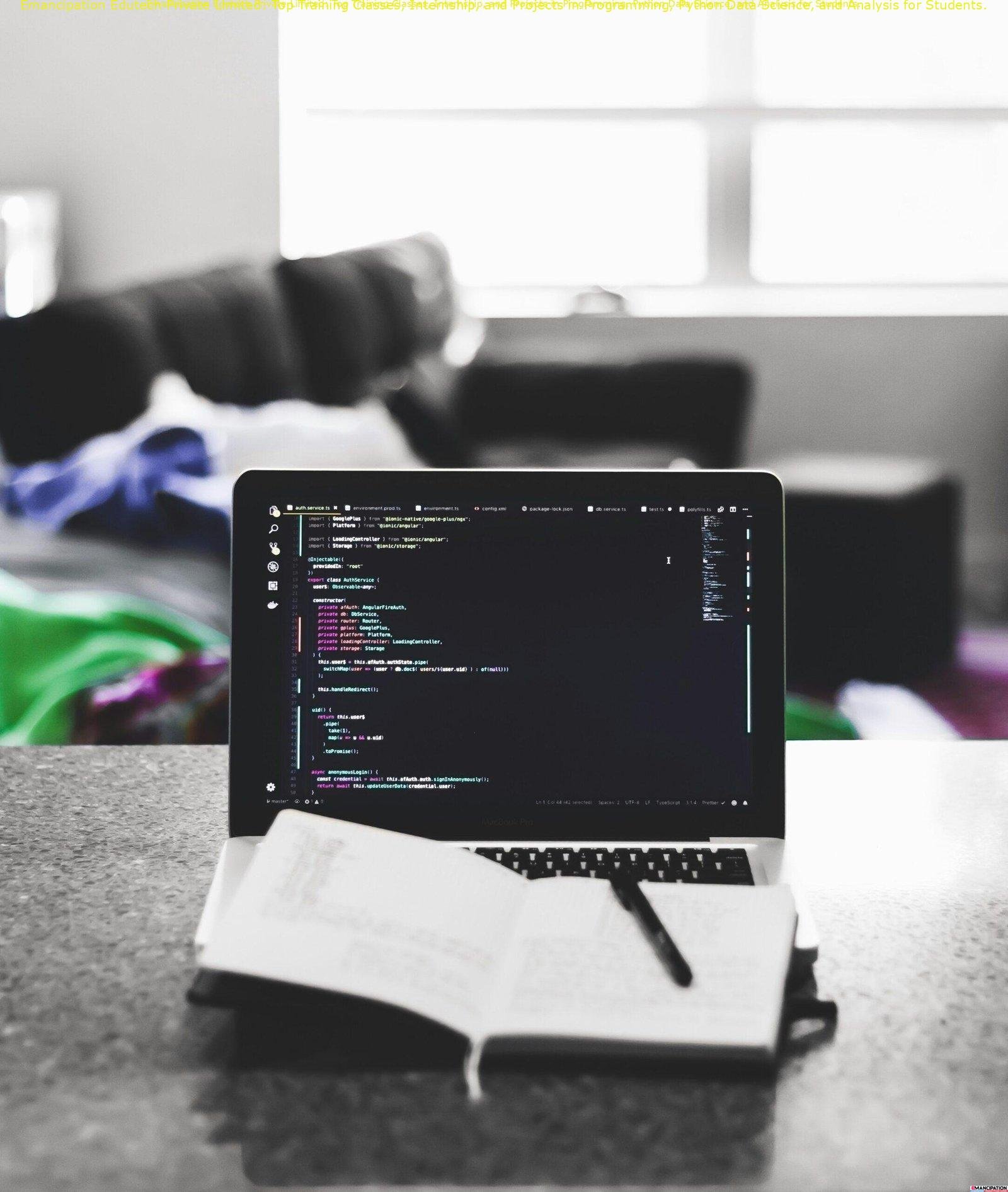
Differences Between Java and Python
Java and Python are both popular programming languages used in various domains of software development. While they share some similarities, such as being object-oriented and having a strong community support, there are also significant differences between the two languages. In this article, we will explore some of the key differences between Java and Python, along with examples to illustrate these differences.
One of the main differences between Java and Python is their syntax. Java uses a statically-typed syntax, which means that variable types must be declared explicitly. For example, if you want to declare an integer variable in Java, you would write:
int num = 10;
On the other hand, Python uses a dynamically-typed syntax, which means that variable types are inferred at runtime. This allows for more flexibility and shorter code. In Python, you can declare the same integer variable without specifying its type:
num = 10
Another difference between Java and Python is their approach to memory management. In Java, memory management is handled automatically by the Java Virtual Machine (JVM) through a process called garbage collection. This means that developers don’t have to worry about deallocating memory manually. However, this can sometimes lead to performance issues and increased memory usage.
On the other hand, Python uses a garbage collector as well, but it also allows developers to manually manage memory through a process called reference counting. This gives developers more control over memory usage and can lead to more efficient code. However, it also requires more attention to memory management and can be more error-prone if not handled properly.
One more important difference between Java and Python is their performance. Java is known for its speed and efficiency, making it a popular choice for high-performance applications. It is compiled into bytecode, which is then executed by the JVM. This allows Java programs to run faster than interpreted languages like Python.
Python, on the other hand, is an interpreted language, which means that it is executed line by line at runtime. This can make Python slower than Java for certain tasks. However, Python has a vast ecosystem of libraries and frameworks that can help optimize performance, making it a versatile language for various applications.
In conclusion, while Java and Python share some similarities, such as being object-oriented and having a strong community support, they also have significant differences in terms of syntax, memory management, and performance. These differences make each language suitable for different use cases, and developers should consider these factors when choosing between Java and Python for their projects.
Another aspect of syntax where Java and Python differ is in their variable declarations. In Java, you need to explicitly declare the data type of a variable before using it. For example, if you want to declare an integer variable named “num” and assign it the value 5, you would write:
int num = 5;
On the other hand, Python is a dynamically typed language, which means that you don’t need to explicitly declare the data type of a variable. You can simply assign a value to a variable, and Python will automatically determine its type. So, to achieve the same result in Python, you would write:
num = 5
This dynamic typing feature of Python makes it more flexible and easier to work with, especially when dealing with complex data structures or when prototyping code quickly. In Java, you would need to specify the data type for each variable, which can be cumbersome and time-consuming.
Furthermore, Java and Python also differ in their approach to handling exceptions. In Java, you need to explicitly catch and handle exceptions using try-catch blocks. This ensures that any potential errors are caught and dealt with appropriately. Here’s an example of how exception handling is done in Java:
try {// code that may throw an exception} catch (Exception e) {// code to handle the exception}
Python, on the other hand, follows a different approach called “Easier to ask for forgiveness than permission” (EAFP). This means that instead of checking for potential errors before executing code, Python assumes that everything will work as expected and catches any exceptions that occur during runtime. Here’s an example of how exception handling is done in Python:
try:# code that may throw an exceptionexcept Exception as e:# code to handle the exception
This difference in exception handling approaches reflects the overall philosophy of the two languages. Java focuses on preventing errors through explicit exception handling, while Python embraces a more flexible and forgiving approach.
In conclusion, the syntax of Java and Python differs significantly. Java has a more verbose syntax with explicit variable declarations and exception handling, while Python has a simpler and more concise syntax. These syntax differences contribute to the overall readability, writability, and flexibility of the two languages.
2. Typing
Another significant difference between Java and Python is their typing system. Java is a statically typed language, which means that variable types need to be declared explicitly and checked at compile-time. Python, on the other hand, is a dynamically typed language, allowing for more flexibility in variable types.
Here’s an example to illustrate the difference:
Java:
int x = 5;String name = "John";boolean flag = true;
Python:
x = 5name = "John"flag = True
In Java, the variable types are explicitly declared, and the compiler will check if the assigned values match the declared types. This strict typing system in Java ensures that the program is free from type-related errors at compile-time, making it more robust and less prone to runtime errors caused by type mismatches.
On the other hand, Python’s dynamically typed nature allows for greater flexibility. Variables can be assigned values of different types without any explicit type declaration. This makes Python more concise and easier to write, as the developer does not have to worry about explicitly specifying the variable type. However, this flexibility comes at a cost – potential type-related errors may only be discovered at runtime, leading to unexpected behavior and bugs.
Both typing systems have their advantages and disadvantages, and the choice between Java and Python often depends on the specific requirements and preferences of the project. If type safety and early error detection are crucial, Java’s static typing may be more suitable. On the other hand, if flexibility and ease of use are prioritized, Python’s dynamic typing may be the preferred choice.